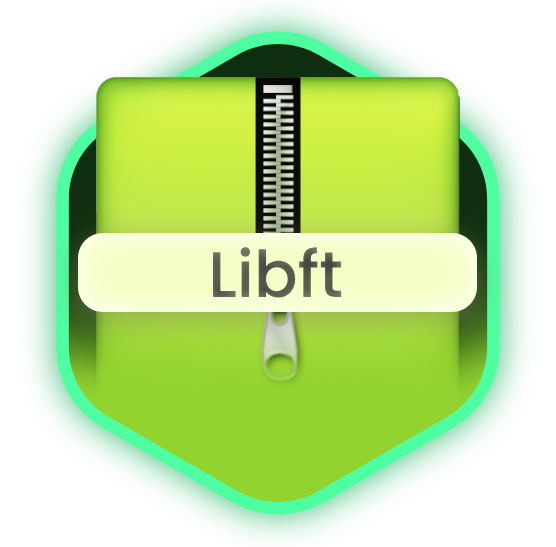
Libft
mrmo7ox
March 21, 2025
Understanding Libft: A Comprehensive Guide
## Table of Contents - [Introduction](#introduction) - [Standard Functions](#standard-functions) - [ft_atoi](#ft_atoi) - [ft_bzero](#ft_bzero) - [ft_calloc](#ft_calloc) - [ft_isalnum](#ft_isalnum) - [ft_isalpha](#ft_isalpha) - [ft_isascii](#ft_isascii) - [ft_isdigit](#ft_isdigit) - [ft_isprint](#ft_isprint) - [ft_itoa](#ft_itoa) - [ft_memchr](#ft_memchr) - [ft_memcmp](#ft_memcmp) - [ft_memcpy](#ft_memcpy) - [ft_memmove](#ft_memmove) - [ft_memset](#ft_memset) - [ft_putchar_fd](#ft_putchar_fd) - [ft_putendl_fd](#ft_putendl_fd) - [ft_putnbr_fd](#ft_putnbr_fd) - [ft_putstr_fd](#ft_putstr_fd) - [ft_split](#ft_split) - [ft_strchr](#ft_strchr) - [ft_strdup](#ft_strdup) - [ft_striteri](#ft_striteri) - [ft_strjoin](#ft_strjoin) - [ft_strlcat](#ft_strlcat) - [ft_strlcpy](#ft_strlcpy) - [ft_strlen](#ft_strlen) - [ft_strmapi](#ft_strmapi) - [ft_strncmp](#ft_strncmp) - [ft_strnstr](#ft_strnstr) - [ft_strrchr](#ft_strrchr) - [ft_strtrim](#ft_strtrim) - [ft_substr](#ft_substr) - [ft_tolower](#ft_tolower) - [ft_toupper](#ft_toupper) - [Bonus Functions](#bonus-functions) - [ft_lstadd_back](#ft_lstadd_back) - [ft_lstadd_front](#ft_lstadd_front) - [ft_lstclear](#ft_lstclear) - [ft_lstdelone](#ft_lstdelone) - [ft_lstiter](#ft_lstiter) - [ft_lstlast](#ft_lstlast) - [ft_lstmap](#ft_lstmap) - [ft_lstnew](#ft_lstnew) - [ft_lstsize](#ft_lstsize) - [Conclusion](#conclusion)
Introduction
Libft is a library that reimplements many of the functions from the C standard library. It includes functions for string manipulation, memory management, and list operations, among others. By implementing these functions, students gain a deeper understanding of how they work and how to use them effectively in their own programs.
ft_atoi
static int overflow (int sign) { if (sign == 1) return (-1); else return (0); } int ft_atoi(const char *str) { unsigned long long result; int sign; unsigned long long max; result = 0; sign = 1; max = 9223372036854775807; while (*str == ' ' || (*str >= 9 && *str <= 13)) str++; if (*str == '+' || *str == '-') { if (*str++ == '-') sign = -1; } while (*str >= '0' && *str <= '9') { if (result > (max - (*str - '0')) / 10) return (overflow(sign)); result = result * 10 + (*str - '0'); str++; } return (result * sign); }
The ft_atoi
function is a custom implementation of the standard C library function atoi
, which converts a string to an integer. Here’s a detailed explanation of how it works:
Helper Function overflow
:
static int overflow (int sign) { if (sign == 1) return (-1); else return (0); }
result
: Stores the intermediate and final integer value.
sign
: Stores the sign of the number (1 for positive, -1 for negative).
max
: Stores the maximum value for a 64-bit signed integer (9223372036854775807
).
- Skipping Whitespace:
while (*str == ' ' || (*str >= 9 && *str <= 13)) str++;
Handling Sign:
if (*str == '+' || *str == '-') { if (*str++ == '-') sign = -1; }
-
- This checks for an optional sign character (
+
or-
). If a-
is found,sign
is set to-1
.
- This checks for an optional sign character (
- Converting Characters to Integer:
while (*str >= '0' && *str <= '9') { if (result > (max - (*str - '0')) / 10) return (overflow(sign)); result = result * 10 + (*str - '0'); str++; }
- This loop processes each digit character (
'0'
to'9'
) and updatesresult
accordingly. - Before updating
result
, it checks for potential overflow. Ifresult
exceeds the threshold where adding another digit would cause overflow, it callsoverflow(sign)
to handle it. - The threshold check is performed using the condition
result > (max - (*str - '0')) / 10
.
ft_isalnum
#include "libft.h" int ft_isalnum(int c) { if (ft_isalpha(c) || ft_isdigit(c)) { return (1); } return (0); }
Parameters
int c: The character to be checked, represented as an integer. This is typically an ASCII value.
Return Value
Returns 1 if the character is alphanumeric (a letter or a digit).
Returns 0 if the character is not alphanumeric.
Implementation Details
Check if the character is a letter or a digit: The function uses two other custom functions, ft_isalpha and ft_isdigit, to check if the character c is a letter or a digit.
ft_isalpha(c): Returns 1 if c is a letter (either lowercase or uppercase), otherwise returns 0.
ft_isdigit(c): Returns 1 if c is a digit (0-9), otherwise returns 0.
Return the result: If either ft_isalpha(c) or ft_isdigit(c) returns 1, the ft_isalnum function returns 1, indicating that the character is alphanumeric. Otherwise, it returns
ft_isalpha
#include "libft.h" int ft_isalpha(int c) { if ((c >= 65 && c <= 90) || (c >= 97 && c <= 122)) { return (1); } return (0); }
Parameters
int c: The character to be checked, represented as an integer. This is typically an ASCII value.
Return Value
Returns 1 if the character is an alphabetic letter (either uppercase or lowercase).
Returns 0 if the character is not an alphabetic letter.
Implementation Details
Check if the character is an uppercase letter: The function first checks if the character c is within the ASCII range for uppercase letters (65 to 90).
c >= 65 && c <= 90: This condition checks if c is between ‘A’ and ‘Z’.
Check if the character is a lowercase letter: If the character is not an uppercase letter, the function then checks if the character c is within the ASCII range for lowercase letters (97 to 122).
c >= 97 && c <= 122: This condition checks if c is between ‘a’ and ‘z’.
Return the result: If either of the above conditions is true, the function returns 1, indicating that the character is an alphabetic letter. Otherwise, it returns 0.
ft_isascii
#include "libft.h" int ft_isascii(int c) { if (c >= 0 && c <= 127) return (1); return (0); }
Parameters
int c: The character to be checked, represented as an integer. This is typically an ASCII value.
Return Value
Returns 1 if the character is an ASCII character.
Returns 0 if the character is not an ASCII character.
Implementation Details
Check if the character is within the ASCII range: The function checks if the character c is within the ASCII range, which is from 0 to 127 inclusive.
c >= 0 && c <= 127: This condition checks if c is between 0 and 127.
Return the result: If the character is within the ASCII range, the function returns 1, indicating that the character is an ASCII character. Otherwise, it returns 0.
ft_isdigit
#include "libft.h" int ft_isdigit(int c) { if (c >= '0' && c <= '9' ) { return (1); } return (0); }
Parameters
int c
: The character to be checked, represented as an integer. This is typically an ASCII value.
Return Value
Returns 1
if the character is a digit (0-9).
Returns 0
if the character is not a digit.
Implementation Details
Check if the character is a digit: The function checks if the character c
is within the ASCII range for digits (48 to 57).
c >= '0' && c <= '9'
: This condition checks if c
is between ‘0’ and ‘9’.
Return the result: If the character is within the range of digits, the function returns 1
, indicating that the character is a digit. Otherwise, it returns 0
.
ft_isprint
int ft_isprint(int c) { if (c >= 32 && c <= 126) return (1); return (0); }
Parameters
int c
: The character to be checked, represented as an integer. This is typically an ASCII value.
Return Value
Returns 1
if the character is a printable character.
Returns 0
if the character is not a printable character.
Implementation Details
Check if the character is within the printable range: The function checks if the character c
is within the ASCII range for printable characters, which is from 32 to 126 inclusive.
c >= 32 && c <= 126
: This condition checks if c
is between 32 (space) and 126 (~
).
Return the result: If the character is within the printable range, the function returns 1
, indicating that the character is printable. Otherwise, it returns 0
.
ft_itoa
#include "libft.h" int get_len(int n) { int i; i = 0; if (n < 0) { i += 1; } while (n) { n /= 10; i++; } return (i); } void put_in(char *p, int n, int len) { int i; i = len - 1; if (n < 0) { p[0] = '-'; n = -n; } while (n > 0) { p[i] = (n % 10) + '0'; n /= 10; i--; } } char *ft_itoa(int n) { int len; char *p; if (n == 0) { p = (char *)malloc(2); if (p) { p[0] = '0'; p[1] = '\0'; } return (p); } if (n == -2147483648) { return (ft_strdup("-2147483648")); } len = get_len(n); p = (char *) malloc(sizeof(char) * (len + 1)); if (!p) return (NULL); put_in (p, n, len); p[len] = '\0'; return (p); }
Helper Functions
Before diving into the implementation of ft_itoa
, let’s first look at the two helper functions: get_len
and put_in
.
get_len
Function
The get_len
function calculates the number of digits in the integer n
, including an extra space for the minus sign if the number is negative.
int get_len(int n) { int i = 0; if (n < 0) { i += 1; } while (n) { n /= 10; i++; } return (i); }
Implementation Details
Initialize the counter: The counter i
is initialized to 0.
Check if the number is negative: If n
is negative, increment the counter by 1 to account for the minus sign.
Calculate the number of digits: Use a while loop to divide n
by 10 until n
becomes zero, incrementing the counter for each division.
put_in
Function
The put_in
function fills the allocated string p
with the digits of the integer n
.
void put_in(char *p, int n, int len) { int i = len - 1; if (n < 0) { p[0] = '-'; n = -n; } while (n > 0) { p[i] = (n % 10) + '0'; n /= 10; i--; } }
Implementation Details
Initialize the index: The index i
is initialized to len - 1
.
Handle negative numbers: If n
is negative, set the first character of p
to '-'
and convert n
to a positive number.
Fill the string with digits: Use a while loop to fill the string p
with digits of n
from the end to the beginning
ft_itoa
Function
The ft_itoa
function converts an integer n
to a null-terminated string.
#include "libft.h" char *ft_itoa(int n) { int len; char *p; if (n == 0) { p = (char *)malloc(2); if (p) { p[0] = '0'; p[1] = '\0'; } return (p); } if (n == -2147483648) { return (ft_strdup("-2147483648")); } len = get_len(n); p = (char *)malloc(sizeof(char) * (len + 1)); if (!p) return (NULL); put_in(p, n, len); p[len] = '\0'; return (p); }
Implementation Details
Handle zero: If n
is zero, allocate memory for a string of length 2, set the first character to '0'
, and the second character to the null terminator.
Handle the minimum integer value: If n
is -2147483648
(the minimum value for a 32-bit signed integer), return the string "-2147483648"
.
Calculate the length of the string: Use get_len
to calculate the length of the string needed to represent n
.
Allocate memory: Allocate memory for the string p
with the calculated length plus one for the null terminator.
Fill the string: Use put_in
to fill the string p
with the digits of n
.
Set the null terminator: Set the last character of the string p
to the null terminator.
Return the string: Return the string p
.
ft_memchr
void *ft_memchr(const void *s, int c, size_t n) { unsigned char *src; unsigned char ch; size_t i; i = 0; src = (unsigned char *)s; ch = (unsigned char)c; while (i < n) { if (src[i] == ch) return ((void *)(&src[i])); i++; } return (NULL); }
Parameters
const void *s
: A pointer to the block of memory where the search will be performed.
int c
: The character to be located, passed as an integer but internally treated as an unsigned char.
size_t n
: The number of bytes to be analyzed in the block of memory.
Return Value
Returns a pointer to the first occurrence of the character in the block of memory.
Returns NULL
if the character is not found within the specified number of bytes.
Implementation Details
Initialize Variables:
src
: A pointer to the block of memory, cast to unsigned char *
for byte-wise operations.
ch
: The character to be located, cast to unsigned char
.
i
: A counter initialized to zero.
Search Loop:
The function uses a while
loop to iterate through the block of memory up to n
bytes.
For each byte, it checks if the current byte (src[i]
) is equal to the target character (ch
).
Return the Result:
If a match is found, the function returns a pointer to the first occurrence of the character in the block of memory.
If no match is found after n
bytes, the function returns NULL
.
ft_memcmp
int ft_memcmp(const void *s1, const void *s2, size_t n) { size_t i; unsigned char *sr1; unsigned char *sr2; sr1 = (unsigned char *) s1; sr2 = (unsigned char *) s2; i = 0; if (n == 0) return (0); while (i < n) { if (sr1[i] != sr2[i]) return (sr1[i] - sr2[i]); i++; } return (0); }
Parameters
const void *s1
: A pointer to the first block of memory to be compared.
const void *s2
: A pointer to the second block of memory to be compared.
size_t n
: The number of bytes to compare.
Return Value
Returns 0
if the two blocks of memory are equal.
Returns a negative value if the first differing byte in s1
is less than the corresponding byte in s2
.
Returns a positive value if the first differing byte in s1
is greater than the corresponding byte in s2
.
Implementation Details
Initialize Variables:
sr1
: A pointer to the first block of memory, cast to unsigned char *
for byte-wise comparison.
sr2
: A pointer to the second block of memory, cast to unsigned char *
.
i
: A counter initialized to zero.
Check for Zero Length:
If n
is zero, the function returns 0
immediately, as there are no bytes to compare.
Comparison Loop:
The function uses a while
loop to iterate through the blocks of memory up to n
bytes.
For each byte, it checks if the corresponding bytes in sr1
and sr2
are different.
Return the Result:
If a difference is found, the function returns the difference between the first differing bytes (sr1[i] - sr2[i]
).
If no difference is found after n
bytes, the function returns 0
.
ft_memcpy
void *ft_memcpy(void *dst, const void *src, size_t n) { unsigned char *tmp_dst; unsigned char *tmp_src; if (dst == (void *)0 && src == (void *)0) return (dst); tmp_dst = (unsigned char *) dst; tmp_src = (unsigned char *) src; while (n > 0) { *tmp_dst++ = *tmp_src++; n--; } return (dst); }
Parameters
void *dst
: A pointer to the destination memory block where the content is to be copied.
const void *src
: A pointer to the source memory block from which the content is to be copied.
size_t n
: The number of bytes to copy from the source to the destination.
Return Value
Returns a pointer to the destination memory block (dst
).
Implementation Details
Check for NULL Pointers:
If both dst
and src
are NULL
, the function returns dst
immediately. This is a safeguard to prevent dereferencing NULL
pointers.
Initialize Temporary Pointers:
tmp_dst
: A pointer to the destination memory block, cast to unsigned char *
for byte-wise operations.
tmp_src
: A pointer to the source memory block, cast to unsigned char *
.
Copy Loop:
The function uses a while
loop to copy n
bytes from the source to the destination memory block.
For each byte, the value at the current position of tmp_src
is copied to the current position of tmp_dst
.
Both pointers are then incremented to move to the next byte, and n
is decremented.
Return the Result:
The function returns the pointer to the destination memory block (dst
).
ft_memmove
void *ft_memmove(void *dest, const void *src, size_t len) { char *new_src; char *new_dst; if (dest == (void *)0 && src == (void *)0) return (NULL); new_src = (char *)src; new_dst = (char *)dest; if (new_dst > new_src) { while (len > 0) { len--; new_dst[len] = new_src[len]; } } else { ft_memcpy (dest, src, len); } return (dest); }
Parameters
void *dest
: A pointer to the destination memory block where the content is to be copied.
const void *src
: A pointer to the source memory block from which the content is to be copied.
size_t len
: The number of bytes to copy from the source to the destination.
Return Value
Returns a pointer to the destination memory block (dest
).
Implementation Details
Check for NULL Pointers:
If both dest
and src
are NULL
, the function returns NULL
immediately. This is a safeguard to prevent dereferencing NULL
pointers.
Initialize Temporary Pointers:
new_src
: A pointer to the source memory block, cast to char *
for byte-wise operations.
new_dst
: A pointer to the destination memory block, cast to char *
.
Handle Overlapping Blocks:
If the destination pointer new_dst
is greater than the source pointer new_src
, the function copies the bytes from the end of the source block to the end of the destination block. This ensures that the source bytes are not overwritten before they are copied.
If there is no overlap, or the source block is after the destination block, the function uses ft_memcpy
to copy the bytes.
Return the Result:
The function returns the pointer to the destination memory block (dest
).
ft_memset
void *ft_memset(void *s, int c, size_t n) { char *new_s; size_t i; i = 0; new_s = (char *)s; while (i < n) { *new_s = c; i++; new_s++; } return (s); }
Parameters
void *s
: A pointer to the block of memory to be filled.
int c
: The value to be set. This is passed as an int
but internally treated as an unsigned char
.
size_t n
: The number of bytes to be set to the value.
Return Value
Returns a pointer to the block of memory (s
).
Implementation Details
Initialize Variables:
new_s
: A pointer to the block of memory, cast to char *
for byte-wise operations.
i
: A counter initialized to zero.
Fill Loop:
The function uses a while
loop to fill n
bytes of the memory block with the value c
.
For each byte, the value c
is assigned to the current position of new_s
.
The pointer new_s
is then incremented to move to the next byte, and i
is incremented.
Return the Result:
The function returns the pointer to the block of memory (s
).
ft_putchar_fd
#include "libft.h" void ft_putchar_fd(char c, int fd) { if (fd < 0) return; write(fd, &c, 1); }
Parameters
char c
: The character to be written.
int fd
: The file descriptor to which the character will be written.
Implementation Details
Check for Invalid File Descriptor:
If the file descriptor fd
is less than 0, the function returns immediately. This is a safeguard to prevent writing to an invalid file descriptor.
Write the Character:
The function uses the write
system call to write the character c
to the specified file descriptor fd
.
ft_putendl_fd
void ft_putendl_fd(char *s, int fd) { int i; if (fd < 0) return ; i = 0; while (s[i]) { write(fd, s + i, 1); i++; } write(fd, "\n", 1); }
Parameters
char *s
: The string to be written.
int fd
: The file descriptor to which the string and newline character will be written.
Implementation Details
Check for Invalid File Descriptor:
If the file descriptor fd
is less than 0, the function returns immediately. This is a safeguard to prevent writing to an invalid file descriptor.
Write the String:
The function uses a while
loop to iterate through each character of the string s
.
For each character, the write
system call is used to write the character to the specified file descriptor fd
.
Write the Newline Character:
After writing the entire string, the function writes a newline character ('\n'
) to the file descriptor
ft_putnbr_fd
void ft_putnbr_fd(int n, int fd) { if (fd < 0) return ; if (n == -2147483648) { ft_putchar_fd('-', fd); ft_putchar_fd('2', fd); n = 147483648; } if (n < 0) { ft_putchar_fd('-', fd); n = -n; } if (n >= 10) { ft_putnbr_fd(n / 10, fd); ft_putchar_fd((n % 10) + '0', fd); } else { ft_putchar_fd(n + '0', fd); } }
Parameters
int n
: The integer to be written.
int fd
: The file descriptor to which the integer will be written.
Implementation Details
The function works as follows:
Check for Invalid File Descriptor:
If the file descriptor fd
is less than 0, the function returns immediately. This is a safeguard to prevent writing to an invalid file descriptor.
Handle the Minimum Integer Value:
If n
is -2147483648
(the minimum value for a 32-bit signed integer), the function handles this special case by writing "-2147483648"
directly to the file descriptor. This is necessary because the absolute value of -2147483648
cannot be represented in a 32-bit signed integer.
Handle Negative Numbers:
If n
is negative, the function writes a '-'
character to the file descriptor and then converts n
to its positive equivalent.
Recursive Writing:
If n
is greater than or equal to 10, the function recursively calls itself with n / 10
to print the higher-order digits.
The function then writes the last digit of n
using ft_putchar_fd
.
Write Single Digit:
If n
is less than 10, the function writes the single digit directly using ft_putchar_fd
.
ft_putstr_fd
void ft_putstr_fd(char *s, int fd) { if (fd < 0 || s == NULL) return ; while (*s) { write(fd, s, 1); s++; } }
Parameters
char *s
: The string to be written.
int fd
: The file descriptor to which the string will be written.
Implementation Details
Check for Invalid File Descriptor or NULL String:
If the file descriptor fd
is less than 0 or the string s
is NULL
, the function returns immediately. This is a safeguard to prevent writing to an invalid file descriptor or dereferencing a NULL
pointer.
Write the String:
The function uses a while
loop to iterate through each character of the string s
.
For each character, the write
system call is used to write the character to the specified file descriptor fd
.
The pointer s
is then incremented to move to the next character.
ft_split
static int get_len(const char *s, char c) { int count; int in_char; count = 0; in_char = 0; while (*s) { if (*s == c && *s) in_char = 0; else if (!in_char) { in_char = 1; count++; } s++; } return (count); } static char **free_n(char **p, int i) { while (i >= 0) { free (p[i]); i--; } free (p); return (NULL); } static char *add_one(const char *s, char c) { char *p; int i; i = 0; while (s[i] != c && s[i]) i++; p = (char *) malloc(sizeof(char) * (i + 1)); if (!p) return (NULL); i = 0; while (*s != c && *s) { p[i] = *s; i++; s++; } p[i] = '\0'; return (p); } char **ft_split(char const *s, char c) { char **p; int len; int i; i = 0; len = get_len(s, c); p = (char **) malloc(sizeof(char *) * (len + 1)); if (!p) return (NULL); while (*s) { while (*s == c && *s) s++; if (*s != c && *s) { p[i] = add_one(s, c); if (p[i] == NULL) return (free_n (p, i)); i++; } while (*s != c && *s) s++; } p[i] = NULL; return (p); }
Parameters
char const *s
: The input string to be split.
char c
: The delimiter character used to split the string.
Return Value
Returns a pointer to an array of strings (substrings) resulting from the split operation.
Returns NULL
if the memory allocation fails.
Implementation Details
Helper Function get_len
:
This static helper function calculates the number of substrings in the input string s
based on the delimiter character c
.
It iterates through the string and counts the number of segments separated by the delimiter.
Helper Function free_n
:
This static helper function frees the memory allocated for the array of strings in case of an error during the split operation.
It iterates through the array of strings and frees each allocated string, then frees the array itself.
Helper Function add_one
:
This static helper function allocates and returns a single substring from the input string s
up to the next occurrence of the delimiter character c
.
It allocates memory for the substring and copies characters from the input string until the delimiter or the end of the string is reached.
Main Function ft_split
:
The main function calculates the number of substrings using get_len
.
It allocates memory for the array of strings based on the calculated length.
It iterates through the input string, skipping delimiter characters and extracting substrings using add_one
.
If memory allocation for a substring fails, it calls free_n
to free all previously allocated memory and returns NULL
.
Finally, it sets the last element of the array to NULL
and returns the array of substrings.
ft_strchr
char *ft_strchr(const char *s, int c) { while (*s) { if (*s == (char)c) return ((char *)s); s++; } if ((char)c == '\0') return ((char *)s); return (NULL); }
Parameters
const char *s
: The input string in which to search for the character.
int c
: The character to be located, passed as an int
but internally treated as a char
.
Return Value
Returns a pointer to the first occurrence of the character c
in the string s
.
Returns NULL
if the character c
is not found in the string.
Implementation Details
Iterate Through the String:
The function uses a while
loop to iterate through each character of the string s
.
For each character, it checks if the character matches the input character c
.
Return the Pointer:
If the character c
is found, the function returns a pointer to the first occurrence of the character in the string.
If the character c
is not found and the loop reaches the null terminator ('\0'
), the function checks if c
is the null terminator. If so, it returns a pointer to the null terminator.
If the character c
is not found and c
is not the null terminator, the function returns NULL
.
ft_strdup
char *ft_strdup(const char *source) { char *dup; int len; len = ft_strlen(source); dup = (char *) malloc(sizeof(char) * (len + 1)); if (dup == NULL) return (NULL); ft_memcpy (dup, source, len); dup[len] = '\0'; return (dup); }
Parameters
const char *source
: The input string to be duplicated.
Return Value
Returns a pointer to the newly allocated string that is a duplicate of the input string.
Returns NULL
if the memory allocation fails.
Implementation Details
Calculate the Length of the Source String:
The function uses ft_strlen
to determine the length of the input string source
.
Allocate Memory for the Duplicate String:
The function allocates memory for the new string using malloc
. The size of the allocated memory is the length of the source string plus one (to accommodate the null terminator).
If the memory allocation fails, the function returns NULL
.
Copy the Source String to the Duplicate String:
The function uses ft_memcpy
to copy the content of the source string into the newly allocated memory.
After copying the content, the function adds a null terminator at the end of the duplicate string.
Return the Duplicate String:
The function returns a pointer to the newly allocated duplicate string.
ft_striteri
void ft_striteri(char *s, void (*f)(unsigned int, char*)) { int i; i = 0; if (s == NULL) return ; while (s[i]) { (*f)(i, &s[i]); i++; } }
Parameters
char *s
: The input string to be iterated over.
void (*f)(unsigned int, char*)
: A function pointer to be applied to each character of the string. The function takes two arguments: the index of the character and a pointer to the character.
Implementation Details
Check for NULL Pointer:
If the input string s
is NULL
, the function returns immediately. This is a safeguard to prevent dereferencing a NULL
pointer.
Iterate Through the String:
The function uses a while
loop to iterate through each character of the string.
For each character, the function calls the function pointed to by f
, passing the current index i
and a pointer to the character &s[i]
as arguments.
Increment Index:
The index i
is incremented in each iteration to move to the next character.
ft_strjoin
char *ft_strjoin(char const *s1, char const *s2) { int len_s1; int len_s2; char *new; if (!s1 && !s2) return (NULL); len_s1 = ft_strlen(s1); len_s2 = ft_strlen(s2); new = (char *)malloc(sizeof(char) * (1 + len_s1 + len_s2)); if (new == NULL) return (NULL); ft_memcpy (new, s1, len_s1); ft_memcpy (new + len_s1, s2, len_s2); new[len_s1 + len_s2] = '\0'; return (new); }
Parameters
char const *s1
: The first input string.
char const *s2
: The second input string.
Return Value
Returns a pointer to the newly allocated string that is the result of concatenating s1
and s2
.
Returns NULL
if the memory allocation fails or if both input strings are NULL
.
Implementation Details
Check for NULL Input Strings:
If both input strings s1
and s2
are NULL
, the function returns NULL
.
Calculate Lengths of Input Strings:
The function uses ft_strlen
to determine the lengths of the input strings s1
and s2
.
Allocate Memory for the New String:
The function allocates memory for the new string using malloc
. The size of the allocated memory is the combined length of s1
and s2
plus one (to accommodate the null terminator).
If the memory allocation fails, the function returns NULL
.
Copy Input Strings to the New String:
The function uses ft_memcpy
to copy the content of s1
into the newly allocated memory.
It then uses ft_memcpy
again to copy the content of s2
immediately after the copied content of s1
.
Add Null Terminator:
After copying the content of both input strings, the function adds a null terminator at the end of the new string.
Return the New String:
The function returns a pointer to the newly allocated concatenated string.
ft_strlcat
#include "libft.h" size_t ft_strlcat(char *dst, const char *src, size_t size) { size_t len_dst; size_t len_src; size_t i; size_t max_copy; len_dst = ft_strlen(dst); len_src = ft_strlen(src); if (size <= len_dst) { return (len_src + size); } max_copy = size - len_dst - 1; i = 0; while (i < max_copy && src[i] != '\0') { dst[len_dst + i] = src[i]; i++; } dst[len_dst + i] = '\0'; return (len_dst + len_src); }
Parameters
char *dst
: The destination buffer to which the string will be appended.
const char *src
: The source string to be appended to the destination buffer.
size_t size
: The total size of the destination buffer, including the null terminator.
Return Value
Returns the total length of the string it tried to create, which is the initial length of dst
plus the length of src
.
Implementation Details
Calculate Lengths of Input Strings:
The function uses ft_strlen
to determine the lengths of the destination string dst
and the source string src
.
Check Buffer Size:
If the size of the buffer is less than or equal to the length of dst
, the function returns the length of src
plus the size of the buffer. This indicates that the buffer size is too small to perform the concatenation.
Calculate Maximum Number of Characters to Copy:
The function calculates the maximum number of characters that can be copied from src
to dst
without exceeding the buffer size. This is given by size - len_dst - 1
.
Copy Characters from Source to Destination:
The function uses a while
loop to copy characters from src
to dst
until it reaches the maximum number of characters to copy or the end of the source string.
Null-Terminate the Result:
After copying the characters, the function adds a null terminator to the end of the destination string.
Return the Total Length:
The function returns the total length of the string it tried to create, which is the sum of the lengths of dst
and src
.
ft_strlcpy
size_t ft_strlcpy(char *dst, const char *src, size_t dstsize) { size_t i; size_t len; i = 0; len = ft_strlen(src); if (dstsize == 0) return (len); while (i < dstsize - 1 && i < len) { dst[i] = src[i]; i++; } dst[i] = '\0'; return (len); }
Parameters
char *dst
: The destination buffer where the string will be copied.
const char *src
: The source string to be copied.
size_t dstsize
: The total size of the destination buffer, including the null terminator.
Return Value
Returns the length of the source string src
.
Implementation Details
Initialize Variables:
The function initializes two variables: i
for indexing and len
to store the length of the source string.
Calculate Length of Source String:
The function uses ft_strlen
to determine the length of the source string src
.
Check Destination Buffer Size:
If the destination buffer size dstsize
is zero, the function returns the length of the source string. This indicates that no characters can be copied.
Copy Characters from Source to Destination:
The function uses a while
loop to copy characters from src
to dst
until it reaches the end of the source string or the destination buffer size minus one (to leave space for the null terminator).
Add Null Terminator:
After copying the characters, the function adds a null terminator to the end of the destination string.
Return the Length of the Source String:
The function returns the length of the source string src
.
ft_strlen
size_t ft_strlen(const char *s) { size_t i; i = 0; while (s[i]) { i++; } return (i); }
Parameters
const char *s
: The input string whose length is to be calculated.
Return Value
Returns the length of the input string s
, excluding the null terminator.
Implementation Details
Initialize Index Variable:
The function initializes a variable i
to 0
. This variable will be used to count the number of characters in the string.
Iterate Through the String:
The function uses a while
loop to iterate through each character of the string s
until it encounters the null terminator ('\0'
).
Increment Index Variable:
For each character in the string, the function increments the variable i
by 1
.
Return the Length:
After the loop completes, the function returns the value of i
, which represents the length of the string.
ft_strmapi
char *ft_strmapi(char const *s, char (*f)(unsigned int, char)) { int i; int len; char *p; if (!s || !f) return (NULL); len = ft_strlen(s) + 1; p = (char *)malloc(sizeof(char) * len); if (p == NULL) return (NULL); i = 0; while (s[i]) { p[i] = f(i, s[i]); i++; } p[i] = 0; return (p); }
Parameters
char const *s
: The input string to be transformed.
char (*f)(unsigned int, char)
: A function pointer that will be applied to each character of the input string. The function takes two arguments: the index of the character and the character itself.
Return Value
Returns a pointer to the newly allocated string that is the result of applying the function f
to each character of the input string s
.
Returns NULL
if the memory allocation fails or if either s
or f
is NULL
.
Implementation Details
Check for NULL Pointers:
If the input string s
or the function pointer f
is NULL
, the function returns NULL
.
Calculate Length of the Input String:
The function uses ft_strlen
to determine the length of the input string s
and adds one to account for the null terminator.
Allocate Memory for the New String:
The function allocates memory for the new string using malloc
. The size of the allocated memory is the length of the input string plus one.
If the memory allocation fails, the function returns NULL
.
Apply the Function to Each Character:
The function uses a while
loop to iterate through each character of the input string s
.
For each character, the function applies f
to the character and its index, and stores the result in the new string p
.
Add Null Terminator:
After processing all characters, the function adds a null terminator to the end of the new string.
Return the New String:
The function returns a pointer to the newly allocated string.
ft_strncmp
int ft_strncmp(const char *s1, const char *s2, size_t n) { size_t i; if (n == 0) return (0); i = 0; while (i < n - 1 && (unsigned char)s1[i] == (unsigned char)s2[i] && s1[i] != '\0' && s2[i] != '\0') { i++; } return ((unsigned char ) s1[i] - (unsigned char) s2[i]); }
Parameters
const char *s1
: The first input string to be compared.
const char *s2
: The second input string to be compared.
size_t n
: The maximum number of characters to compare.
Return Value
Returns an integer less than, equal to, or greater than zero if the first n
characters of s1
are found, respectively, to be less than, to match, or be greater than the first n
characters of s2
.
Implementation Details
Check for Zero Length:
If n
is zero, the function returns 0
immediately. This indicates that no characters are compared.
Initialize Index Variable:
The function initializes a variable i
to 0
. This variable will be used to iterate through the characters of the strings.
Iterate and Compare Characters:
The function uses a while
loop to iterate through the characters of the strings s1
and s2
.
The loop continues as long as i
is less than n - 1
, the characters at the current index in both strings are equal, and neither character is the null terminator ('\0'
).
Return the Difference:
After the loop completes, the function returns the difference between the characters at the current index in both strings, cast to unsigned char
.
ft_strnstr
char *ft_strnstr(const char *big, const char *little, size_t len) { size_t i; size_t j; i = 0; if (*little == '\0') return ((char *)big); if (len == 0) return (NULL); while (big[i] && i < len) { if (len - i < ft_strlen(little)) return (NULL); j = 0; while (little[j] && (i + j) < len && big[i + j] == little[j]) { j++; } if (little[j] == '\0') return ((char *)(big + i)); i++; } return (NULL); }
Parameters
const char *big
: The larger string in which to search for the substring.
const char *little
: The substring to search for within the larger string.
size_t len
: The maximum number of characters to search within the larger string.
Return Value
Returns a pointer to the first occurrence of the substring little
in the string big
, limited to len
characters.
Returns NULL
if the substring is not found within the specified range.
If the little
string is empty, the function returns big
.
Implementation Details
Initialize Variables:
The function initializes two variables: i
for indexing through big
and j
for indexing through little
.
Check for Empty Substring:
If the little
string is empty, the function returns big
immediately.
Iterate Through the Larger String:
The function uses a while
loop to iterate through the big
string up to len
characters.
For each character in big
, it checks if there is enough space left to match the little
string. If not, it returns NULL
.
Compare Substrings:
The function uses a nested while
loop to compare characters of big
and little
.
If all characters of little
match a substring in big
, the function returns a pointer to the start of the matching substring in big
.
Return Result:
If no match is found after iterating through big
, the function returns NULL
.
ft_strrchr
char *ft_strrchr(const char *s, int c) { const char *last_occurrence = NULL; while (*s) { if (*s == (char)c) last_occurrence = s; s++; } if ((char)c == '\0') return ((char *)s); return ((char *)last_occurrence); }
Parameters
const char *s
: The input string in which to search for the character.
int c
: The character to be located, passed as an int
but internally treated as a char
.
Return Value
Returns a pointer to the last occurrence of the character c
in the string s
.
Returns NULL
if the character c
is not found in the string.
Implementation Details
The function works as follows:
Initialize Last Occurrence Pointer:
The function initializes a pointer last_occurrence
to NULL
. This pointer will be used to keep track of the last occurrence of the character c
in the string s
.
Iterate Through the String:
The function uses a while
loop to iterate through each character of the string s
.
For each character, it checks if the character matches the input character c
.
If a match is found, the function updates the last_occurrence
pointer to point to the current character.
Check for Null Terminator:
After the loop completes, the function checks if the input character c
is the null terminator ('\0'
). If so, it returns a pointer to the null terminator at the end of the string s
.
Return the Last Occurrence Pointer:
The function returns the last_occurrence
pointer, which points to the last occurrence of the character c
in the string s
.
If the character c
is not found, the function returns NULL
ft_strtrim
int to_remove(const char *set, char c) { while (*set) { if (*set == c) return (1); set++; } return (0); } char *ft_allocpy(const char *p, size_t start, size_t end) { size_t total; char *new; total = end - start; new = (char *)malloc(total + 1); if (!new) return (NULL); ft_memcpy(new, p + start, total); new[total] = '\0'; return (new); } char *len_trim(const char *s1, const char *set) { size_t i; size_t j; i = 0; j = ft_strlen(s1); while (to_remove(set, s1[i]) && s1[i] != '\0') i++; while (to_remove(set, s1[j - 1]) && j > i) j--; if (i >= j) return (ft_allocpy("", 0, 0)); return (ft_allocpy(s1, i, j)); } char *ft_strtrim(char const *s1, char const *set) { if (!s1) return (NULL); return (len_trim(s1, set)); }
Parameters
const char *set
: The set of characters to check against.
char c
: The character to check for in the set.
Return Value
Returns 1
if the character c
is found in the set.
Returns 0
otherwise.
Implementation Details
The function iterates through the set of characters and returns 1
if it finds the character c
. If it reaches the end of the set without finding the character, it returns 0
.
ft_allocpy
Helper Function
The ft_allocpy
function allocates memory and copies a portion of the input string.
char *ft_allocpy(const char *p, size_t start, size_t end);
Parameters
const char *p
: The input string to copy from.
size_t start
: The starting index of the portion to copy.
size_t end
: The ending index (exclusive) of the portion to copy.
Return Value
Returns a pointer to the newly allocated string containing the copied portion.
Returns NULL
if memory allocation fails.
Implementation Details
The function calculates the total length of the portion to copy and allocates memory for it. It then copies the specified portion of the input string into the newly allocated memory and adds a null terminator at the end.
len_trim
Helper Function
The len_trim
function finds the start and end indices for trimming the input string.
char *len_trim(const char *s1, const char *set);
Parameters
const char *s1
: The input string to be trimmed.
const char *set
: The set of characters to remove from the input string.
Return Value
Returns a pointer to the newly allocated trimmed string.
Implementation Details
The function iterates through the input string from both the start and the end to find the indices where the trimming should begin and end. It then calls ft_allocpy
to allocate memory and copy the trimmed portion of the input string.
ft_strtrim
Function
The ft_strtrim
function removes leading and trailing characters from the input string.
char *ft_strtrim(char const *s1, char const *set);
Parameters
char const *s1
: The input string to be trimmed.
char const *set
: The set of characters to remove from the input string.
Return Value
Returns a pointer to the newly allocated trimmed string.
Returns NULL
if the input string is NULL
.
Implementation Details
The function checks if the input string is NULL
and returns NULL
if it is. Otherwise, it calls the len_trim
function to perform the trimming and return the result.
ft_substr
char *ft_substr(char const *s, unsigned int start, size_t len) { char *sub; size_t s_len; s_len = ft_strlen(s); if (!s) return (NULL); if (start >= s_len) return (ft_strdup("")); if (len > s_len - start) len = s_len - start; sub = (char *) malloc(sizeof(char) * (len + 1)); if (sub == NULL) return (NULL); ft_memcpy(sub, s + start, len); sub[len] = '\0'; return (sub); }
Parameters
char const *s
: The input string from which the substring will be extracted.
unsigned int start
: The starting index from which to begin the extraction.
size_t len
: The maximum length of the substring to be extracted.
Return Value
Returns a pointer to the newly allocated substring.
Returns NULL
if the memory allocation fails or if the input string s
is NULL
.
Implementation Details
Calculate Length of Input String:
The function uses ft_strlen
to determine the length of the input string s
.
Check for NULL Input String:
If the input string s
is NULL
, the function returns NULL
.
Check Starting Index:
If the starting index start
is greater than or equal to the length of the input string, the function returns an empty string by calling ft_strdup("")
.
Adjust Length if Necessary:
If the specified length len
is greater than the remaining length of the input string from the starting index, the function adjusts len
to be the remaining length.
Allocate Memory for the Substring:
The function allocates memory for the substring using malloc
. The size of the allocated memory is len + 1
to accommodate the null terminator.
If the memory allocation fails, the function returns NULL
.
Copy the Substring:
The function uses ft_memcpy
to copy the specified portion of the input string into the newly allocated memory.
Add Null Terminator:
The function adds a null terminator to the end of the substring.
Return the Substring:
The function returns a pointer to the newly allocated substring.
ft_tolower
int ft_tolower(int c) { if (c >= 65 && c <= 90) { return (c + 32); } return (c); }
Parameters
int c
: The character to be converted, passed as an integer.
Return Value
Returns the lowercase equivalent of the character if it is an uppercase letter.
Returns the character unchanged if it is not an uppercase letter.
Implementation Details
Check if the Character is Uppercase:
The function checks if the character c
is within the ASCII range for uppercase letters (65 to 90 inclusive).
Convert to Lowercase:
If c
is an uppercase letter, the function converts it to the corresponding lowercase letter by adding 32 to its ASCII value.
Return the Character:
The function returns the converted character if it was an uppercase letter, or the original character if it was not.
ft_toupper
int ft_toupper(int c) { if (c >= 97 && c <= 122) { return (c - 32); } return (c); }
Parameters
int c
: The character to be converted, passed as an integer.
Return Value
Returns the uppercase equivalent of the character if it is a lowercase letter.
Returns the character unchanged if it is not a lowercase letter.
Implementation Details
Check if the Character is Lowercase:
The function checks if the character c
is within the ASCII range for lowercase letters (97 to 122 inclusive).
Convert to Uppercase:
If c
is a lowercase letter, the function converts it to the corresponding uppercase letter by subtracting 32 from its ASCII value.
Return the Character:
The function returns the converted character if it was a lowercase letter, or the original character if it was not.
ft_lstadd_back
void ft_lstadd_back(t_list **lst, t_list *new) { t_list *last; if (new == NULL || lst == NULL) return ; if (*lst == NULL) { *lst = new; return ; } last = ft_lstlast(*lst); last->next = new; }
Parameters
t_list **lst
: A pointer to the first element of the linked list.
t_list *new
: The new element to be added to the end of the linked list.
Return Value
The function does not return a value.
Implementation Details
Check for NULL Pointers:
If the new element new
or the list lst
is NULL
, the function returns immediately.
Check if the List is Empty:
If the list *lst
is NULL
, the new element new
becomes the first element of the list.
The function sets *lst
to new
and returns.
Find the Last Element:
The function uses ft_lstlast
to find the last element of the linked list.
Add the New Element to the End:
The function sets the next
pointer of the last element to the new element new
.
ft_lstadd_front
void ft_lstadd_front(t_list **lst, t_list *new) { if (lst == NULL || new == NULL) return ; new -> next = *lst; *lst = new; }
Parameters
t_list **lst
: A pointer to the first element of the linked list.
t_list *new
: The new element to be added to the beginning of the linked list.
Return Value
The function does not return a value.
Implementation Details
Check for NULL Pointers:
If the new element new
or the list lst
is NULL
, the function returns immediately.
Set the Next Pointer of New Element:
The function sets the next
pointer of the new element new
to point to the current first element of the list *lst
.
Update the Head of the List:
The function updates the head of the list to point to the new element new
.
ft_lstclear
void ft_lstclear(t_list **lst, void (*del)(void*)) { t_list *on; t_list *next; if (!(*lst) && !del) return ; on = *lst; while (on) { next = on -> next; del(on -> content); free(on); on = next; } *lst = (NULL); }
Parameters
t_list **lst
: A pointer to the first element of the linked list.
void (*del)(void*)
: A pointer to a function used to delete the content of each element.
Return Value
The function does not return a value.
Implementation Details
Check for NULL Pointers:
If the list lst
is NULL
or the delete function del
is NULL
, the function returns immediately.
Initialize Pointers:
The function initializes two pointers: on
to iterate through the list and next
to store the next element.
Iterate Through the List:
The function uses a while
loop to iterate through each element of the list.
Delete and Free Each Element:
For each element, the function:
Stores the next element in next
.
Uses the del
function to delete the content of the current element.
Frees the memory of the current element.
Moves to the next element using the next
pointer.
Set the List Pointer to NULL:
After all elements have been deleted and freed, the function sets the list pointer *lst
to NULL
.
ft_lstdelone
void ft_lstdelone(t_list *lst, void (*del)(void *)) { if (lst && del) { del(lst->content); free (lst); } }
Parameters
t_list *lst
: The element to be deleted.
void (*del)(void *)
: A pointer to a function used to delete the content of the element.
Return Value
The function does not return a value.
Implementation Details
Check for NULL Pointers:
If the element lst
or the delete function del
is NULL
, the function returns immediately.
Delete the Content:
The function uses the del
function to delete the content of the element lst
.
Free the Element:
The function frees the memory of the element lst
.
ft_lstiter
void ft_lstiter(t_list *lst, void (*f)(void *)) { if (!(lst || f)) return ; while (lst) { f(lst->content); lst = lst->next; } }
Parameters
t_list *lst
: The first element of the linked list.
void (*f)(void *)
: A pointer to a function that takes a single argument (the content of each element) and returns nothing.
Return Value
The function does not return a value.
Implementation Details
Check for NULL Pointers:
If the list lst
or the function f
is NULL
, the function returns immediately.
Iterate Through the List:
The function uses a while
loop to iterate over each element of the list.
Apply Function to Each Element:
For each element, the function applies the specified function f
to the content of the element.
Move to the Next Element:
The function moves to the next element in the list using the next
pointer.
ft_lstlast
t_list *ft_lstlast(t_list *lst) { if (lst == NULL) return (NULL); while (lst->next != NULL) { lst = lst->next; } return (lst); }
Parameters
t_list *lst
: The first element of the linked list.
Return Value
Returns a pointer to the last element of the linked list.
Returns NULL
if the list is empty.
Implementation Details
Check if the List is Empty:
If the list lst
is NULL
, the function returns NULL
.
Iterate Through the List:
The function uses a while
loop to iterate through each element of the list until it reaches the last element (where next
is NULL
).
Return the Last Element:
The function returns a pointer to the last element of the list.
static void handle_error(t_list **head, void *contenty, void (*del)(void *)) { ft_lstclear (head, del); if (contenty) del (contenty); } t_list *ft_lstmap(t_list *lst, void *(*f)(void *), void (*del)(void *)) { t_list *head; t_list *node; void *contenty; if (!lst || !f || !del) return (NULL); head = NULL; while (lst) { contenty = f(lst->content); if (!contenty) { handle_error(&head, contenty, del); } node = ft_lstnew(contenty); if (!node) { handle_error(&head, contenty, del); } ft_lstadd_back (&head, node); lst = lst->next; } return (head); }
Parameters
t_list *lst
: The first element of the linked list to be mapped.
void *(*f)(void *)
: A pointer to a function that takes a single argument (the content of each element) and returns a new content.
void (*del)(void *)
: A pointer to a function that takes a single argument (the content of an element) and frees it.
Return Value
Returns a pointer to the first element of the new linked list.
Returns NULL
if memory allocation fails or if any of the input parameters are NULL
.
Implementation Details
Check for NULL Parameters:
If the list lst
, the function f
, or the delete function del
is NULL
, the function returns NULL
.
Initialize Head of New List:
The function initializes a pointer head
to NULL
to keep track of the new list.
Iterate Through the List:
The function uses a while
loop to iterate over each element of the original list.
Apply Function and Create New Node:
For each element, the function applies the function f
to the content of the element.
If f
returns NULL
, the handle_error
function is called to clean up and the function returns NULL
.
Otherwise, a new node is created using ft_lstnew
. If memory allocation fails, the handle_error
function is called to clean up and the function returns NULL
.
Add New Node to New List:
The function adds the new node to the end of the new list using ft_lstadd_back
.
Return the New List:
After processing all elements, the function returns the head of the new list.
ft_lstnew
t_list *ft_lstnew(void *content) { struct s_list *new; new = (struct s_list *) malloc(sizeof(struct s_list)); if (new == NULL) return (NULL); new -> content = content; new -> next = NULL; return (new); }
Parameters
void *content
: The content to be stored in the new element of the linked list.
Return Value
Returns a pointer to the newly created element.
Returns NULL
if memory allocation fails.
Implementation Details
Allocate Memory for New Element:
The function allocates memory for the new element using malloc
. The size of the allocated memory is the size of the t_list
structure.
Check for Memory Allocation Failure:
If the memory allocation fails, the function returns NULL
.
Initialize the New Element:
The function initializes the content
field of the new element with the provided content
.
The function initializes the next
field of the new element to NULL
.
Return the New Element:
The function returns a pointer to the newly created element.
ft_lstsize
int ft_lstsize(t_list *lst) { int i; if (lst == NULL) return (0); i = 0; while (lst) { i++; lst = lst->next; } return (i); }
Parameters
t_list *lst
: The first element of the linked list.
Return Value
Returns the number of elements in the linked list.
Returns 0
if the list is empty.
Implementation Details
Check if the List is Empty:
If the list lst
is NULL
, the function returns 0
.
Initialize Counter:
The function initializes an integer i
to 0
to keep track of the number of elements.
Iterate Through the List:
The function uses a while
loop to iterate through each element of the list.
For each iteration, the function increments the counter i
by 1
.
Return the Counter:
After processing all elements, the function returns the counter i
, which represents the number of elements in the list.
#ifndef LIBFT_H # define LIBFT_H # include <unistd.h> # include <stdlib.h> int ft_atoi(const char *ptr); void ft_bzero(void *s, size_t n); void *ft_calloc(size_t number, size_t size); int ft_isalnum(int c); int ft_isalpha(int c); int ft_isascii(int c); int ft_isdigit(int c); int ft_isprint(int c); char *ft_itoa(int n); void *ft_memchr(const void *s, int c, size_t n); int ft_memcmp(const void *s1, const void *s2, size_t n); void *ft_memcpy(void *dst, const void *src, size_t n); void *ft_memmove(void *dest, const void *src, size_t len); void *ft_memset(void *s, int c, size_t n); void ft_putchar_fd(char c, int fd); void ft_putendl_fd(char *s, int fd); void ft_putnbr_fd(int n, int fd); void ft_putstr_fd(char *s, int fd); char **ft_split(char const *s, char c); char *ft_strchr(const char *s, int c); char *ft_strdup(const char *source); void ft_striteri(char *s, void (*f)(unsigned int, char*)); char *ft_strjoin(char const *s1, char const *s2); size_t ft_strlcat(char *dst, const char *src, size_t size); size_t ft_strlcpy(char *dst, const char *src, size_t dstsize); size_t ft_strlen(const char *s); char *ft_strmapi(char const *s, char (*f)(unsigned int, char)); int ft_strncmp(const char *s1, const char *s2, size_t n); char *ft_strnstr(const char *big, const char *little, size_t len); char *ft_strrchr(const char *s, int c); char *ft_strtrim(char const *s1, char const *set); char *ft_substr(char const *s, unsigned int start, size_t len); int ft_tolower(int c); int ft_toupper(int c); typedef struct s_list { void *content; struct s_list *next; } t_list; t_list *ft_lstnew(void *content); void ft_lstadd_front(t_list **lst, t_list *new); int ft_lstsize(t_list *lst); t_list *ft_lstlast(t_list *lst); void ft_lstadd_back(t_list **lst, t_list *new); void ft_lstdelone(t_list *lst, void (*del)(void *)); void ft_lstclear(t_list **lst, void (*del)(void *)); void ft_lstiter(t_list *lst, void (*f)(void *)); t_list *ft_lstmap(t_list *lst, void *(*f)(void *), void (*del)(void *)); #endif
Include Syntax: <>
vs. ""
#include <...>
: This syntax is used for including standard library headers. The angle brackets instruct the preprocessor to look for the header file in the system directories where standard libraries are stored.
#include "..."
: This syntax is used for including user-defined or local header files. The double quotes instruct the preprocessor to look for the header file in the current directory first, and if it’s not found, then in the system directories.
How File Guards Work
Define a Unique Identifier:
A unique identifier is defined using #define
preprocessor directive. This identifier is usually based on the file name to ensure uniqueness.
Check if the Identifier is Already Defined:
The #ifndef
(if not defined) preprocessor directive checks if the unique identifier is already defined. If it is not defined, the code inside the guard is included.
Define the Identifier:
If the identifier is not defined, it is defined using the #define
preprocessor directive. This prevents the header file from being included again in the same compilation.
End the Guard:
The #endif
Makefile
CC = cc CFLAGS = -Wall -Wextra -Werror SRCS = ft_atoi.c ft_bzero.c ft_calloc.c ft_isalnum.c ft_isalpha.c ft_isascii.c \ ft_isdigit.c ft_isprint.c ft_itoa.c ft_memchr.c ft_memcmp.c ft_memcpy.c \ ft_memmove.c ft_memset.c ft_putchar_fd.c ft_putendl_fd.c ft_putnbr_fd.c \ ft_putstr_fd.c ft_split.c ft_strchr.c ft_strdup.c ft_striteri.c ft_strjoin.c \ ft_strlcat.c ft_strlcpy.c ft_strlen.c ft_strmapi.c ft_strncmp.c ft_strnstr.c \ ft_strrchr.c ft_strtrim.c ft_substr.c ft_tolower.c ft_toupper.c BONUS = ft_lstadd_back_bonus.c ft_lstadd_front_bonus.c ft_lstclear_bonus.c \ ft_lstdelone_bonus.c ft_lstiter_bonus.c ft_lstlast_bonus.c ft_lstmap_bonus.c \ ft_lstnew_bonus.c ft_lstsize_bonus.c OBJECTS = $(SRCS:.c=.o) B_OBJS = $(BONUS:.c=.o) NAME = libft.a all: $(NAME) $(NAME): $(OBJECTS) ar rcs $@ $^ %.o: %.c libft.h $(CC) $(CFLAGS) -c $< -o $@ bonus: $(B_OBJS) ar rcs $(NAME) $^ clean: rm -f $(OBJECTS) $(B_OBJS) fclean: clean rm -f $(NAME) re: fclean all .PHONY: clean
Makefile
Explanation
A Makefile
is a script used by the make
build automation tool to compile and manage projects. In this case, the Makefile
is designed for compiling the libft
library, which consists of various C source files.
Variables
CC = cc
Specifies the compiler to be used. In this case, it is set to cc
.
CFLAGS:
CFLAGS = -Wall -Wextra -Werror
Specifies the compiler flags. These flags enable all warnings (-Wall
), extra warnings (-Wextra
), and treat all warnings as errors (-Werror
).
SRCS:
SRCS = ft_atoi.c ft_bzero.c ft_calloc.c ft_isalnum.c ft_isalpha.c ft_isascii.c
ft_isdigit.c ft_isprint.c ft_itoa.c ft_memchr.c ft_memcmp.c ft_memcpy.c \
ft_memmove.c ft_memset.c ft_putchar_fd.c ft_putendl_fd.c ft_putnbr_fd.c \
ft_putstr_fd.c ft_split.c ft_strchr.c ft_strdup.c ft_striteri.c ft_strjoin.c \
ft_strlcat.c ft_strlcpy.c ft_strlen.c ft_strmapi.c ft_strncmp.c ft_strnstr.c \
ft_strrchr.c ft_strtrim.c ft_substr.c ft_tolower.c ft_toupper.c
BONUS:
BONUS = ft_lstadd_back_bonus.c ft_lstadd_front_bonus.c ft_lstclear_bonus.c \
ft_lstdelone_bonus.c ft_lstiter_bonus.c ft_lstlast_bonus.c ft_lstmap_bonus.c \
ft_lstnew_bonus.c ft_lstsize_bonus.c
Lists the source files for the bonus part of the library, which typically includes functions for linked list operations.
OBJECTS
OBJECTS = $(SRCS:.c=.o)
Lists the object files corresponding to the main source files.
B_OBJS:
B_OBJS = $(BONUS:.c=.o)
Lists the object files corresponding to the bonus source files.
NAME:
NAME = libft.a
Specifies the name of the output library file.
all:
all: $(NAME)
The default target, which depends on the $(NAME)
target. Running make
without arguments will build this target.
$(NAME):
$(NAME): $(OBJECTS)
ar rcs $@ $^
Builds the library file $(NAME)
from the object files $(OBJECTS)
. The ar rcs
command creates an archive (static library) and adds the object files to it.
Object File Compilation:
%.o: %.c libft.h
$(CC) $(CFLAGS) -c $< -o $@
Compiles each .c
file into a .o
object file. The $<
represents the source file, and $@
represents the target object file.
bonus:
bonus: $(B_OBJS)
ar rcs $(NAME) $^
Builds the bonus object files and adds them to the library file $(NAME)
.
clean:
clean:
rm -f $(OBJECTS) $(B_OBJS)
Removes the compiled object files.
fclean:
fclean: clean
rm -f $(NAME)
Removes the compiled object files and the library file.
re:
re: fclean all
Rebuilds the entire project by first cleaning all compiled files and then building the default target.
.PHONY:
Declares clean
as a phony target, meaning it is not associated with files of the same name and will always be executed when invoked.